NOTE:This is a read only archive of threads posted to the FreeRTOS support forum. Use these archive pages to search previous posts. New forum support threads can be started at the
FreeRTOS forums.
FreeRTOS Support Archive
The FreeRTOS support forum can be used for active support both from Amazon Web Services and the community. In return for using our software for free, we request you play fair and do your bit to help others! Sign up for an account and receive notifications of new support topics then help where you can.
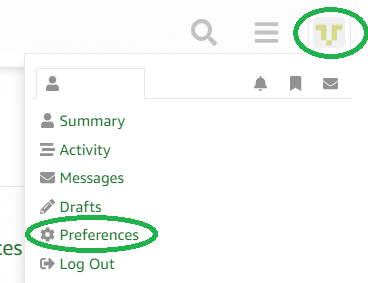
This is a read only archive of threads posted to the FreeRTOS support forum. Use these archive pages to search previous posts. New forum support threads can be started at the FreeRTOS forums.
[FreeRTOS Home]
[Live FreeRTOS Forum]
[FAQ]
[Archive Top]
[January 2019 Threads]
Retrieve the size and maximum usage of the stack per task
Posted by
mastupristi on January 30, 2019
For reasons related to debugging our application we use the function
uxTaskGetSystemState()
. It populates a vector of structures
TaskStatus_t
. In this structure is also present the field
usStackHighWaterMark
, whose comment says:
/ * The minimum amount of stack space that has remained for the task since the task was created. The closer this value is to zero the closer the task has come to overflowing its stack. * /
For the same reasons of debugging, we also need to know the size of the stack, and the maximum usage percentage of it.
How can I get this information with the functions and structures available?
best regards
Max
Retrieve the size and maximum usage of the stack per task
Posted by
rtel on January 30, 2019
If you set configRECORDSTACKHIGH_ADDRESS to 1 in FreeRTOSConfig.h then
the other end of the stack is also recorded in the task’s TCB –
depending on which version of FreeRTOS you are using (its quite a new
feature), but I don’t think there is a function to retrieve it so you
would have to look in the TCB manually.
However, as the size of a task’s stack is defined by the application
writer when the task is created I would suggest you already know the
total size of the stack.
Retrieve the size and maximum usage of the stack per task
Posted by
mastupristi on January 30, 2019
It would be better if we could get the information from the process structures, because it’s true that I know the information when I create tasks, but it’s also true that in the moment in which the vector of TaskStatus_t
is populated it’s difficult, if not impossible, to access such information.
However, I use version 10.0.1
Retrieve the size and maximum usage of the stack per task
Posted by
rtel on January 30, 2019
If you look at the bottom of tasks.c you will see that, with the correct
defines, you can insert your own code into tasks.c by providing header
files that are included into the c file directly. That enables any
extensions you want for your project without the need to edit the
kernel’s source files.
Retrieve the size and maximum usage of the stack per task
Posted by
mastupristi on January 30, 2019
I did as you say, and I was successful, thank you.
Is this patch considering itself an addition to FreeRTOS? Do I have to share the code?
best regards
Retrieve the size and maximum usage of the stack per task
Posted by
rtel on January 30, 2019
It’s always nice when people share code, but you don’t have to.
Retrieve the size and maximum usage of the stack per task
Posted by
mastupristi on January 31, 2019
Here’s the patch. I tried to follow the FreeRTOS coding rules, but I’m not sure I did it all.
~~~
diff –git a/FW/amazon-freertos/lib/FreeRTOS/tasks.c b/FW/amazon-freertos/lib/FreeRTOS/tasks.c
index 20c3962..6393e8f 100644
— a/FW/amazon-freertos/lib/FreeRTOS/tasks.c
+++ b/FW/amazon-freertos/lib/FreeRTOS/tasks.c
@@ -5042,4 +5042,27 @@ when performing module tests). */
#endif
+#if( ( FREERTOSADDITIONALFEATURETOTALSTACKAPI == 1 ) && ( configRECORDSTACKHIGHADDRESS == 1 ) )
+
+uint32t uxTaskGetStackSize( TaskHandlet xTask )
+{
+ uint32t uxReturn;
+ TCBt *pxTCB;
+
+ if( xTask != NULL )
+ {
+ pxTCB = ( TCB_t * ) xTask;
+ uxReturn = (pxTCB->pxEndOfStack – pxTCB->pxStack) * sizeof(StackType_t);
+ }
+ else
+ {
+ uxReturn = 0;
+ }
+
+ return uxReturn;
+}
+
+#endif
diff –git a/FW/amazon-freertos/lib/include/task.h b/FW/amazon-freertos/lib/include/task.h
index d53b4dd..2a36eef 100644
— a/FW/amazon-freertos/lib/include/task.h
+++ b/FW/amazon-freertos/lib/include/task.h
@@ -2359,6 +2359,12 @@ void *pvTaskIncrementMutexHeldCount( void ) PRIVILEGED_FUNCTION;
void vTaskInternalSetTimeOutState( TimeOut_t * const pxTimeOut ) PRIVILEGED_FUNCTION;
+#if( ( FREERTOSADDITIONALFEATURETOTALSTACKAPI == 1 ) && ( configRECORDSTACKHIGHADDRESS == 1 ) )
+
+uint32t uxTaskGetStackSize( TaskHandlet xTask );
+
+#endif
+
#ifdef __cplusplus
}
#endif
~~~
To enable this function is necessary that also the symbol FREERTOS_ADDITIONAL_FEATURE_TOTAL_STACK_API
is defined to 1. I do it on the command line when I launch make.
best regards
Max
Copyright (C) Amazon Web Services, Inc. or its affiliates. All rights reserved.